Here’s how we can create a simple pop-up window via the UIAlertView instance. It can have a title, some info text and several buttons (OK, Cancel, etc). We’ll only deal with one button and not worry about how to read out which button value has been pressed.
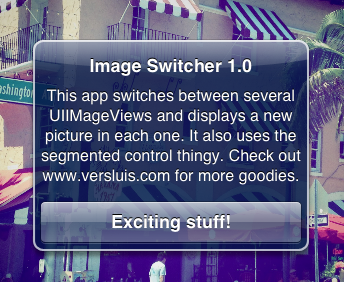
This is what a UIAlertView looks like
And here’s how we build this on Objective-C:
UIAlertView *infoMessage; infoMessage = [[UIAlertView alloc] initWithTitle:@"Image Switcher 1.0" message:@"This app switches between several UIIMageViews and displays a new picture in each one. It also uses the segmented control thingy. Check out http://www.versluis.com for more goodies." delegate:self cancelButtonTitle:@"Exciting stuff!" otherButtonTitles:nil]; infoMessage.alertViewStyle = UIAlertViewStyleDefault; [infoMessage show];
This is quite a long block of code so here are the steps:
- create an instance of the UIAlertView
- allocate and initiate it with parameters Title, Message, and an array of buttons (cancel and nil if you’re only using a single button to dismiss the view)
- give the view a style (mandatory)
- display the view
You can add a \n for a line break inside the window.
Here is a shorter version of the code, hopefully more cut-and-paste friendly: